class: center, middle, inverse, title-slide # Zonebuilders ## Cross-platform and language-agnostic tools for generating zoning systems for urban analysis and modelling ### Robin Lovelace, Martijn Tennekes, Dustin Carlino, FOSS4G 2021 --- ## About us .left-column[ ```r # Reproducible # R code # Install pkgs... library(tmap) library(tidyverse) tmap_mode("view") people = c("Robin", "Martijn", "Dustin") location = c("Leeds, UK", "Maastricht, Holland", "Seattle, USA") year = c(2019, 2019, 2020) people_locations = tmaptools::geocode_OSM(location, as.sf = TRUE) people_data_frame = data.frame(people, location, year) people_sf = sf::st_sf(people_data_frame, geometry = people_locations$point) zones = purrr::map_dfr(location, zonebuilder::zb_zone) m = tm_shape(zones) + tm_borders() + tm_text("label", col = "grey") + tm_shape(people_sf) + tm_text("people", scale = 2) ``` ] .right-column[
] --- .left-column[ # Why? - Every city has a different zoning system - Need for consistency when doing inter-city comparisons - Need for a general solution - Existing 'zone-building' systems start with 'basic statistical units' (BSUs) - Fun FOSS programming challenge! ] -- .right-column[ ## Available zones are often arbitrary
] ??? - Contents: ... <!-- --- --> <!-- # Early prototypes --> --- #### Application: communicating and navigating city locations  ??? Illustration of how the ClockBoard system could be used to describe the approximate location of places. In this hypothetical example, someone is describing key places to visit in and around Leeds to someone who arrives at the train station in zone A, visits the city’s famous Roundhay Park in zone C01, before travelling for an evening meal in Bradford, in zone E09. --- ## Application: exploring city scale data  --- background-image: url(https://github.com/zonebuilders/zonebuilder/releases/download/v0.0.2.9000/cities_p1.png) background-position: center background-size: contain -- #### Inter-city #### comparisons #### population --- background-image: url(https://zonebuilders.github.io/zonebuilder/articles/paper_files/figure-html/intercity-1.png) background-size: contain #### Application: inter-city comparisons: urban phenomena --- # Using the ZoneBuilder software .pull-left[ ```r library(zonebuilder) z = zb_zone( "Buenos Aires", n_circles = 9 ) ``` ```r library(tmap) tmap_mode("view") tm_shape(z) + tm_borders() + tm_basemap(leaflet::providers$OpenStreetMap) + tm_scale_bar() ``` ] .pull-right[
] --- ## With the R package You can install the released version of zonebuilder from [CRAN](https://CRAN.R-project.org) with: ```r install.packages("zonebuilder") ``` Install it from [GitHub](https://github.com/) with: ```r # install.packages("remotes") remotes::install_github("zonebuilders/zonebuilder") ``` ```r library(zonebuilder) library(tmap) tm_shape(london_a()) + tm_borders() + tm_shape(london_c()) + tm_dots("red") ```
--- ## Create zones in one command ```r london_zones <- zb_zone(london_c(), london_a()) zb_plot(london_zones) ``` <img src="index_files/figure-html/unnamed-chunk-10-1.png" width="100%" /> --- # Output the results ```r sf::write_sf(london_zones, "london_zones.geojson") ``` --- ## With the Rust crate ### See https://github.com/zonebuilders/zonebuilder-rust Install Rust + cargo ```bash cargo install zonebuilder ``` See the help with: ```bash zonebuilder -h ``` ``` ## zb 0.1.0 ## Configures a clockboard diagram ## ## USAGE: ## zonebuilder [FLAGS] [OPTIONS] ## ## FLAGS: ## -h, --help Prints help information ## --projected Is the data projected? ## -V, --version Prints version information ## ## OPTIONS: ## -d, --distances <distances>... ## The distances between concentric rings. `triangular_sequence` is useful to generate these distances ## [default: 1.0,3.0,6.0,10.0,15.0] ## -s, --num-segments <num-segments> ## The number of radial segments. Defaults to 12, like the hours on a clock [default: 12] ## ## -v, --num-vertices-arc <num-vertices-arc> ## The number of vertices per arc. Higher values approximate a circle more accurately [default: 10] ## ## -p, --precision <precision> ## The number of decimal places in the resulting output GeoJSON files. Set to 6 by default. Larger numbers mean ## more precision, but larger file sizes [default: 6] ``` --- ## Minimal Rust example .pull-left[ ```bash zonebuilder -s 3 -d 1.0,3.0 > zones.geojson cat zones.geojson ``` ``` ## { ## "features": [ ## { ## "geometry": { ## "coordinates": [ ## [ ## [ ## 0.0, ## 0.009043 ## ], ## [ ## 0.001867, ## 0.008846 ## ], ## [ ## 0.003653, ## 0.008261 ## ], ## [ ## 0.00528, ## 0.007316 ## ], ## [ ## 0.006675, ## 0.006051 ## ], ## [ ## 0.007779, ## 0.004521 ## ], ## [ ## 0.008543, ## 0.002794 ## ], ## [ ## 0.008933, ## 0.000945 ## ], ## [ ## 0.008933, ## -0.000945 ## ], ## [ ## 0.008543, ## -0.002794 ## ], ## [ ## 0.007779, ## -0.004521 ## ], ## [ ## 0.006675, ## -0.006051 ## ], ## [ ## 0.00528, ## -0.007316 ## ], ## [ ## 0.003653, ## -0.008261 ## ], ## [ ## 0.001867, ## -0.008846 ## ], ## [ ## 0.0, ## -0.009043 ## ], ## [ ## -0.001867, ## -0.008846 ## ], ## [ ## -0.003653, ## -0.008261 ## ], ## [ ## -0.00528, ## -0.007316 ## ], ## [ ## -0.006675, ## -0.006051 ## ], ## [ ## -0.007779, ## -0.004521 ## ], ## [ ## -0.008543, ## -0.002794 ## ], ## [ ## -0.008933, ## -0.000945 ## ], ## [ ## -0.008933, ## 0.000945 ## ], ## [ ## -0.008543, ## 0.002794 ## ], ## [ ## -0.007779, ## 0.004521 ## ], ## [ ## -0.006675, ## 0.006051 ## ], ## [ ## -0.00528, ## 0.007316 ## ], ## [ ## -0.003653, ## 0.008261 ## ], ## [ ## -0.001867, ## 0.008846 ## ], ## [ ## 0.0, ## 0.009043 ## ] ## ] ## ], ## "type": "Polygon" ## }, ## "properties": { ## "label": "A" ## }, ## "type": "Feature" ## }, ## { ## "geometry": { ## "coordinates": [ ## [ ## [ ## 0.023338, ## 0.013565 ## ], ## [ ## 0.02563, ## 0.008383 ## ], ## [ ## 0.026801, ## 0.002835 ## ], ## [ ## 0.026801, ## -0.002835 ## ], ## [ ## 0.02563, ## -0.008383 ## ], ## [ ## 0.023338, ## -0.013565 ## ], ## [ ## 0.020027, ## -0.018154 ## ], ## [ ## 0.01584, ## -0.021949 ## ], ## [ ## 0.010961, ## -0.024785 ## ], ## [ ## 0.005603, ## -0.026538 ## ], ## [ ## 0.0, ## -0.027131 ## ], ## [ ## 0.0, ## -0.009043 ## ], ## [ ## 0.001867, ## -0.008846 ## ], ## [ ## 0.003653, ## -0.008261 ## ], ## [ ## 0.00528, ## -0.007316 ## ], ## [ ## 0.006675, ## -0.006051 ## ], ## [ ## 0.007779, ## -0.004521 ## ], ## [ ## 0.008543, ## -0.002794 ## ], ## [ ## 0.008933, ## -0.000945 ## ], ## [ ## 0.008933, ## 0.000945 ## ], ## [ ## 0.008543, ## 0.002794 ## ], ## [ ## 0.007779, ## 0.004521 ## ], ## [ ## 0.023338, ## 0.013565 ## ] ## ] ## ], ## "type": "Polygon" ## }, ## "properties": { ## "label": "B01" ## }, ## "type": "Feature" ## }, ## { ## "geometry": { ## "coordinates": [ ## [ ## [ ## 0.0, ## -0.027131 ## ], ## [ ## -0.005603, ## -0.026538 ## ], ## [ ## -0.010961, ## -0.024785 ## ], ## [ ## -0.01584, ## -0.021949 ## ], ## [ ## -0.020027, ## -0.018154 ## ], ## [ ## -0.023338, ## -0.013565 ## ], ## [ ## -0.02563, ## -0.008383 ## ], ## [ ## -0.026801, ## -0.002835 ## ], ## [ ## -0.026801, ## 0.002835 ## ], ## [ ## -0.02563, ## 0.008383 ## ], ## [ ## -0.023338, ## 0.013565 ## ], ## [ ## -0.007779, ## 0.004521 ## ], ## [ ## -0.008543, ## 0.002794 ## ], ## [ ## -0.008933, ## 0.000945 ## ], ## [ ## -0.008933, ## -0.000945 ## ], ## [ ## -0.008543, ## -0.002794 ## ], ## [ ## -0.007779, ## -0.004521 ## ], ## [ ## -0.006675, ## -0.006051 ## ], ## [ ## -0.00528, ## -0.007316 ## ], ## [ ## -0.003653, ## -0.008261 ## ], ## [ ## -0.001867, ## -0.008846 ## ], ## [ ## 0.0, ## -0.009043 ## ], ## [ ## 0.0, ## -0.027131 ## ] ## ] ## ], ## "type": "Polygon" ## }, ## "properties": { ## "label": "B02" ## }, ## "type": "Feature" ## }, ## { ## "geometry": { ## "coordinates": [ ## [ ## [ ## -0.023338, ## 0.013565 ## ], ## [ ## -0.020027, ## 0.018154 ## ], ## [ ## -0.01584, ## 0.021949 ## ], ## [ ## -0.010961, ## 0.024785 ## ], ## [ ## -0.005603, ## 0.026538 ## ], ## [ ## 0.0, ## 0.027131 ## ], ## [ ## 0.005603, ## 0.026538 ## ], ## [ ## 0.010961, ## 0.024785 ## ], ## [ ## 0.01584, ## 0.021949 ## ], ## [ ## 0.020027, ## 0.018154 ## ], ## [ ## 0.023338, ## 0.013565 ## ], ## [ ## 0.007779, ## 0.004521 ## ], ## [ ## 0.006675, ## 0.006051 ## ], ## [ ## 0.00528, ## 0.007316 ## ], ## [ ## 0.003653, ## 0.008261 ## ], ## [ ## 0.001867, ## 0.008846 ## ], ## [ ## 0.0, ## 0.009043 ## ], ## [ ## -0.001867, ## 0.008846 ## ], ## [ ## -0.003653, ## 0.008261 ## ], ## [ ## -0.00528, ## 0.007316 ## ], ## [ ## -0.006675, ## 0.006051 ## ], ## [ ## -0.007779, ## 0.004521 ## ], ## [ ## -0.023338, ## 0.013565 ## ] ## ] ## ], ## "type": "Polygon" ## }, ## "properties": { ## "label": "B03" ## }, ## "type": "Feature" ## } ## ], ## "type": "FeatureCollection" ## } ``` ] .pull-right[  ] --- ## With the Python package or QGIS plugin 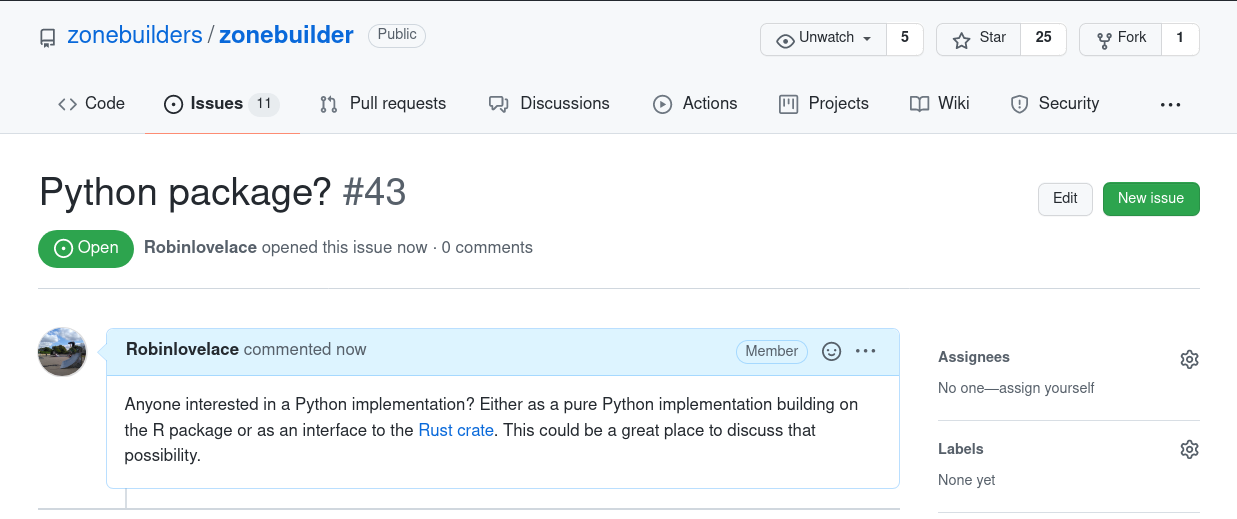 -- See https://github.com/zonebuilders/zonebuilder/issues/41 ??? Let us know if you'd like to get involved! --- ## In your web browser 🤯 Try the Wasm based tool at: https://zonebuilders.github.io/zonebuilder-rust/ 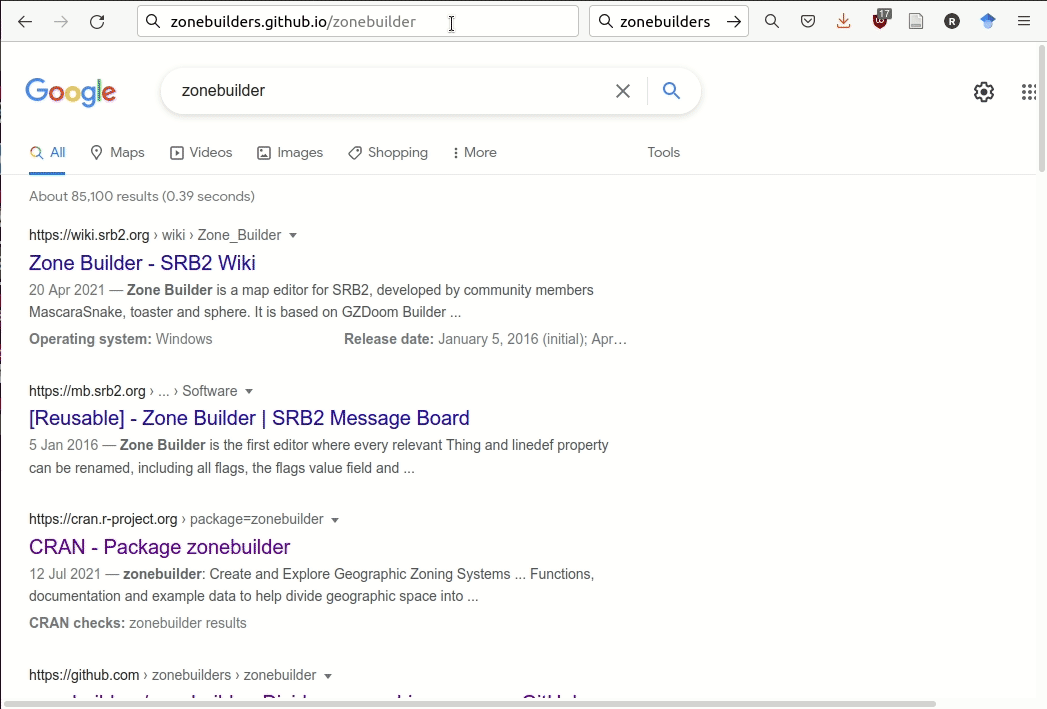 --- # The ClockBoard paper - Preprint paper on the ClockBoard zoning system. See https://doi.org/10.31219/osf.io/vncgw for details 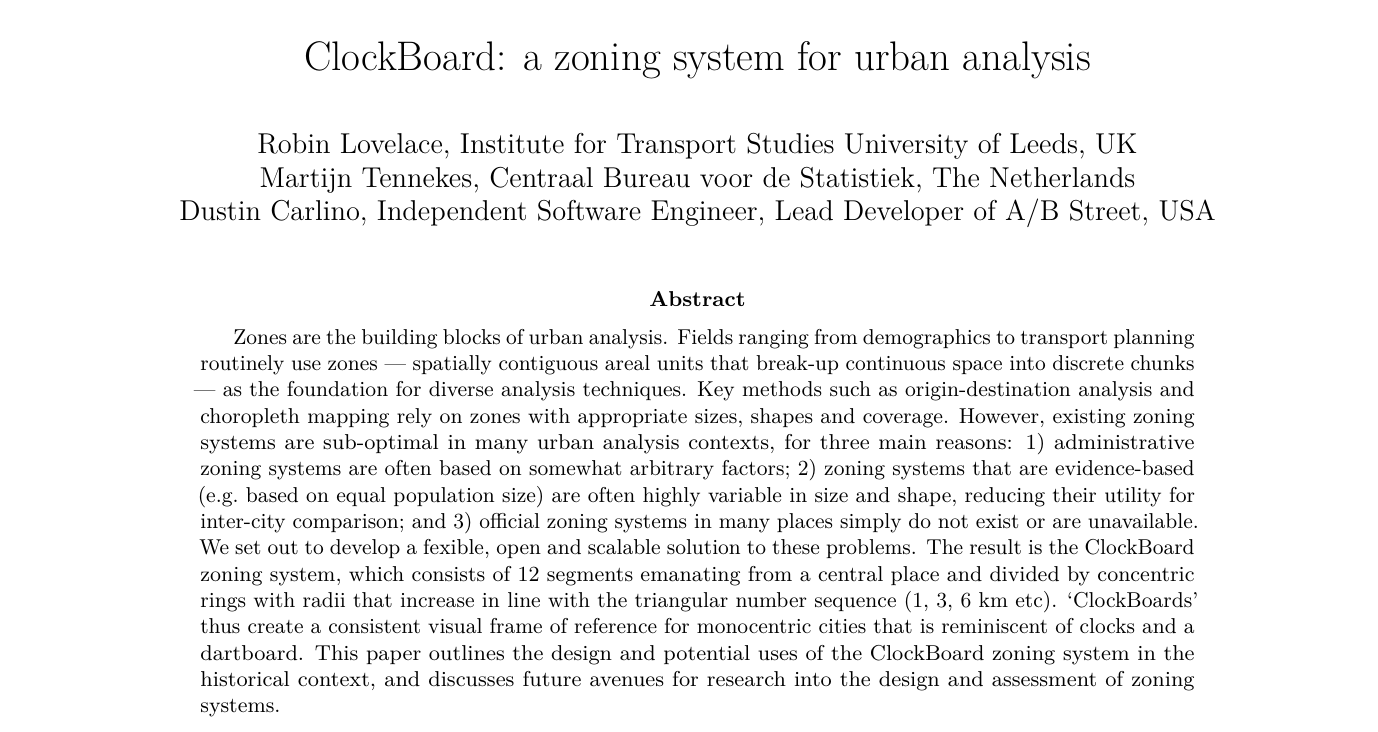 --- # Next steps .pull-left[ ## Conceptually - How much can the ClockBoard concept catch on? - What alternative zoning systems can be imagined/created? - How to design a zoning system incorporating multiple city centres? ] .pull-right[ ## Technologically - Fix any outstanding bugs in R and Rust implementations - Add features to R and Rust implemenations - Zonebuilder implementations in other languages - Python, JavaScript, Julia, Go? Great geo learning exercise. - Interfaces to the Rust implementation, e.g. via [rextendr](https://github.com/extendr/rextendr) R package and the [pyo3](https://github.com/PyO3/pyo3) Python package - Create standard zones ready to be used for major cities worldwide ] --- # Thanks - Stephan Hügel and the GeoRust community for support with the Rust code - The broadly defined R-Spatial community for making the R package possible - The OSGEO community and developers for making solid open source software possible ## Links - [Paper](https://zonebuilders.github.io/zonebuilder/articles/paper.html) describing the ClockBoard zoning system, implemented with zonebuilders - [zonebuilder R package](https://zonebuilders.github.io/zonebuilder) for building zones in R - [zonebuilder Rust crate](https://crates.io/crates/zonebuilder) for building zones in Rust/anywhere - [zonebuilder web app](https://zonebuilders.github.io/zonebuilder-rust) for building zones in your browser - [Reproducible source code](https://github.com/zonebuilders/zonebuilders.github.io/blob/master/index.Rmd) of these slides (and the software) on GitHub